Creating Custom Validators In Reactive Forms Using Angular 6
Blog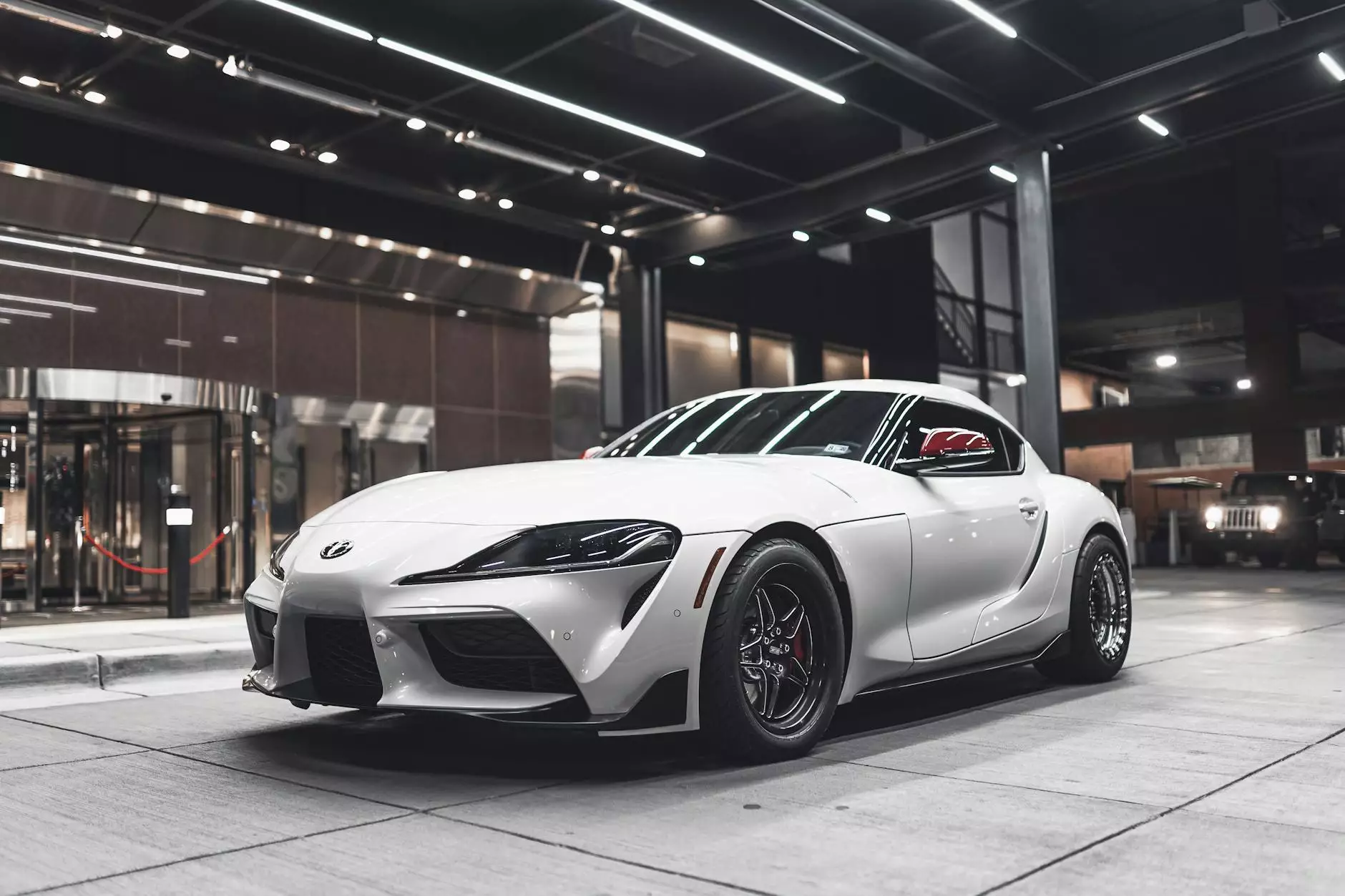
Welcome to SEO Tycoon, a leading provider of SEO services in the DFW area. We specialize in web design and marketing to help businesses improve their online presence. In this article, we will explore the process of creating custom validators in Angular 6 for reactive forms.
Why Custom Validators?
Validators play a crucial role in ensuring the data entered in forms meets specific requirements. While Angular provides a set of built-in validators, there are cases when custom validators are necessary to meet unique business needs. By creating custom validators, you can enforce your own validation rules and enhance the user experience.
Understanding Reactive Forms
Before diving into custom validators, it's essential to have an understanding of reactive forms in Angular. Reactive forms offer a powerful way to build dynamic and complex forms with ease. They provide greater control over validation, asynchronous operations, and form data manipulation.
Step-by-Step Guide: Creating Custom Validators
1. Setting Up the Environment
To begin, make sure you have Angular 6 installed on your system. If not, you can easily set it up by following the official Angular documentation. Once Angular is installed, create a new Angular project using the Angular CLI.
2. Creating a Custom Validator
Next, let's create a custom validator by extending the Validator interface provided by Angular. This interface requires the implementation of a validate method that accepts a FormControl object and returns a validation result.
import { AbstractControl, ValidatorFn } from '@angular/forms'; export function customValidator(): ValidatorFn { return (control: AbstractControl): { [key: string]: any } | null => { // Your validation logic here // Return an object with the error code if validation fails, else return null }; }Within the validate method, you can define your custom validation logic. Return an object with the error code if the validation fails, or return null if it passes. This way, you can provide appropriate error messages to the user.
3. Adding the Custom Validator to a Reactive Form
Now that we have our custom validator, we can use it in our reactive form. To add the custom validator to a form control, we can pass it as a second parameter to the Validators array provided by Angular.
import { Component } from '@angular/core'; import { FormBuilder, FormGroup, Validators } from '@angular/forms'; import { customValidator } from './custom-validator'; @Component({ selector: 'app-custom-form', templateUrl: './custom-form.component.html', styleUrls: ['./custom-form.component.css'] }) export class CustomFormComponent { customForm: FormGroup; constructor(private fb: FormBuilder) { this.customForm = this.fb.group({ email: ['', [Validators.required, customValidator()]], // Add more form controls here }); } // Other form-related methods here }In the example above, we've added the custom validator to the "email" form control. You can add additional form controls and apply the custom validator on them as needed.
4. Enhancing User Experience
Lastly, to provide a better user experience, you can display meaningful error messages when the custom validator fails. You can achieve this by accessing the form control's error object in your HTML template.
Email is required. Email is not valid.In the code snippet above, we utilize Angular's built-in directives to display error messages to the user based on the validation results.
Conclusion
Congratulations! You have successfully learned how to create custom validators in Angular 6 for reactive forms. Custom validators are a powerful tool to enforce unique validation rules in your applications. At SEO Tycoon, we understand the importance of a robust online presence for businesses. Our expert team offers top-notch SEO services, including web design and marketing, to help you achieve your digital goals. Contact us today to supercharge your online presence!